Continuous Integration / Continuous Deployment (CI/CD) frameworks lift your code to professional standards of collaboration, rapid iteration, and frequent deployment. Experiments can either succeed or fail and revert seamlessly. Small, testable changes can keep your code base stable and adherent to Service-Level Agreements (SLAs).
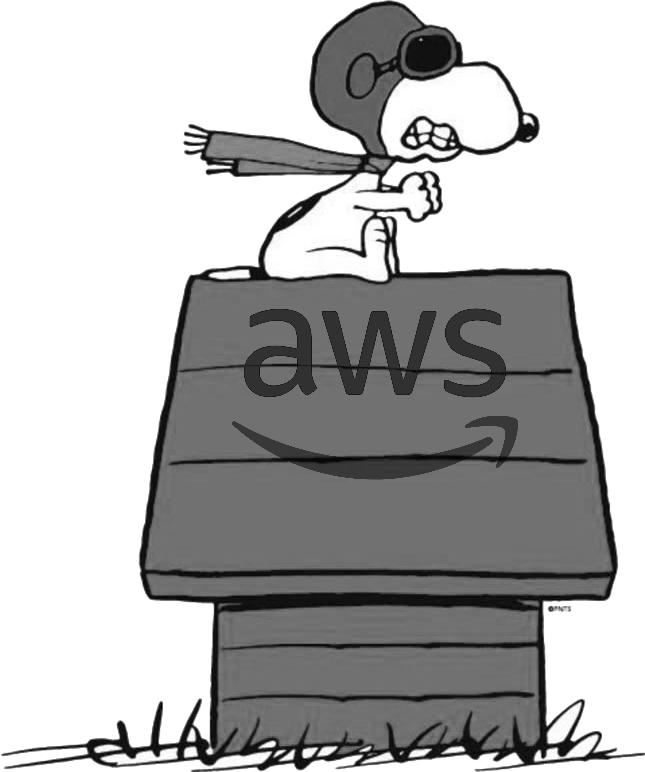
Unlike Data Science and Data Analysis, Web Development often starts as a hobby. After the blissful experience of building a website, and watching it behave expectedly while running locally, then next step is to deploy your site for the world’s amazement.
At one time this meant buying space a web server (often through a hosting platform like GoDaddy or BlueHost) and using an FTP to move your files onto a running platform. Et voila, the site has now left the nest. It is live. If bugs appear in production, the site code you corrected the code, and redeployed the files via FTP. This process is inevitably difficult to iterate upon and “version”, i.e. to be certain what code was actually live in production. CI/CD frameworks provide the conventions and resources to make the process more deliberate, secure, and ultimately faster.
The role of the Application Developer often overlaps Dev Ops. Application code is deployed continuously and in collaboration with other developers. Teams develop at a rapidly with frequent changes. Managers and clients want visibility into these changes. Software packages need to be deployed in multiple environments, such as Development and Production, with small modifications between them. At this point stability is a must. Infrastructure as Code (IaC) is the gyroscope to stabilize your ship. Within the Amazon Web Services (AWS) landscape, AWS CloudFormation, AWS Cloud Development Kit (CDK) and AWS Serverless Application Model (SAM) are your best bets. These frameworks are an exoskeleton for your app. For traditional websites AWS Amplify Gen 2 is a new garage/laboratory to keep your code healthy and innovative.
AWS CloudFormation defines the resources needed to deploy your app. Besides referencing application code, the templates can specify networking and compute resources need to run the app in the cloud. CloudFormation templates are written as either JSON or YAML and can be deployed through the AWS Console or with the AWS Command Line Interface (CLI). The following code, saved as datajammers.yaml
represents a CloudFormation template:
AWSTemplateFormatVersion: 2010-09-09
Description: Template for Webserver with Security Group and EC2 Instance
Parameters:
LatestAmiId:
Description: The latest Amazon Linux 2 AMI from the Parameter Store
Type: AWS::SSM::Parameter::Value<AWS::EC2::Image::Id>
Default: /aws/service/ami-amazon-linux-latest/amzn2-ami-hvm-x86_64-gp2
InstanceType:
Description: Webserver EC2 instance type
Type: String
Default: t2.micro
AllowedValues:
- t3.micro
- t2.micro
ConstraintDescription: must be a valid EC2 instance type.
MyIP:
Description: Your IP address in CIDR format
Type: String
MinLength: 9
MaxLength: 18
AllowedPattern: (\d{1,3})\.(\d{1,3})\.(\d{1,3})\.(\d{1,3})/(\d{1,2})
Default: 0.0.0.0/0
AllowedPatter: '^(\d{1,3}\.){3}\d{1,3}\/\d{1,2}$'
ConstraintDescription: Must be a valid IP CIDR range of the form x.x.x.x/x
Resources:
WebServerSecurityGroup:
Type: AWS::EC2::SecurityGroup
Properties:
GroupDescription: Allow HTTP access via my IP address
SecurityGroupIngress:
- IpProtocol: tcp
FromPort: 80
ToPort: 80
CidrIp: !Ref MyIP
- IpProtocol: tcp
WebServer:
Type: AWS::EC2::Instance
Properties:
ImageId: !Ref LatestAmiId
InstanceType: !Ref InstanceType
SecurityGroups:
- !Ref WebServerSecurityGroup
UserData: !Base64 |
#!/bin/bash
yum update -y
yum install -y httpd
systemctl start httpd
systemctl enable httpd
echo "<html><body><h1>Data Jammers!</h1></body></html>" > /var/www/html/index.html
Outputs:
WebsiteURL:
Value: !Join
- ""
- - http://
- !GetAtt WebServer.PublicDnsName
Description: WebsiteURL
This template deploys a stack with an EC2 instance, WebServer
. The instance UserData loads a webserver, which displays a Data Jammers!
message when the website loads. The server has a security group, WebServerSecurityGroup
which allows HTTP traffic only from the specified ip (defaulting to 0.0.0.0/0
– the IPv4 CIDR range which includes every possible IP address). The stack has one output, WebsiteURL
, the URL of deployed website.
To deploy this stack from a local laptop using the aws-cli, run the following command:
aws cloudformation create-stack --stack-name DataJammers --template-body file://datajammers.yaml --region us-east-1
In the CloudFormation panel of the AWS console in the us-east-1
region the output of the DataJammers
stack has an output listing the WebsiteURL
of the deployed website.
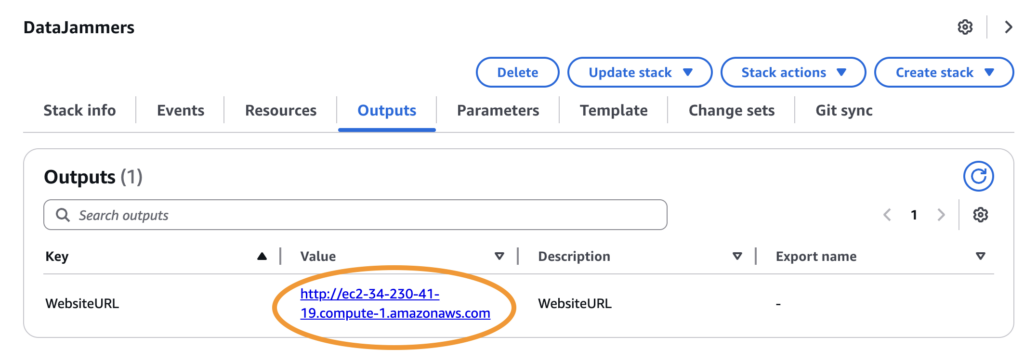
Loading the WebsiteURL
in a browser pulls up the welcome message.
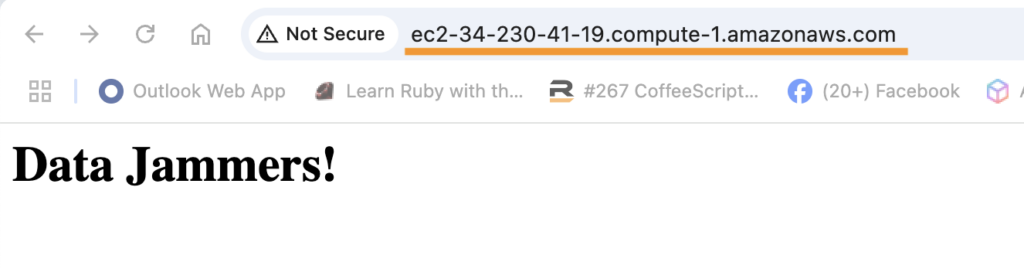
Not that this browser page loads via http
(not https
) over port 80. To enable https for security, an SSL certifcate group can be provisioned with AWS Certificate Manager, and traffic must be allowed on port 443.
AWS Cloud Develoment Kit (CDK)
AWS Cloud Development Kit (CDK) is the next layer of abstraction. CDK is a preprocessor framework, which can be implemented in many languages, including Typescript, JavaScript, Python, Java, C#/.Net, and Go. These scripts compile to produce CloudFormation templates. Typescript ensures strong-typing, thus reducing runtime errors.
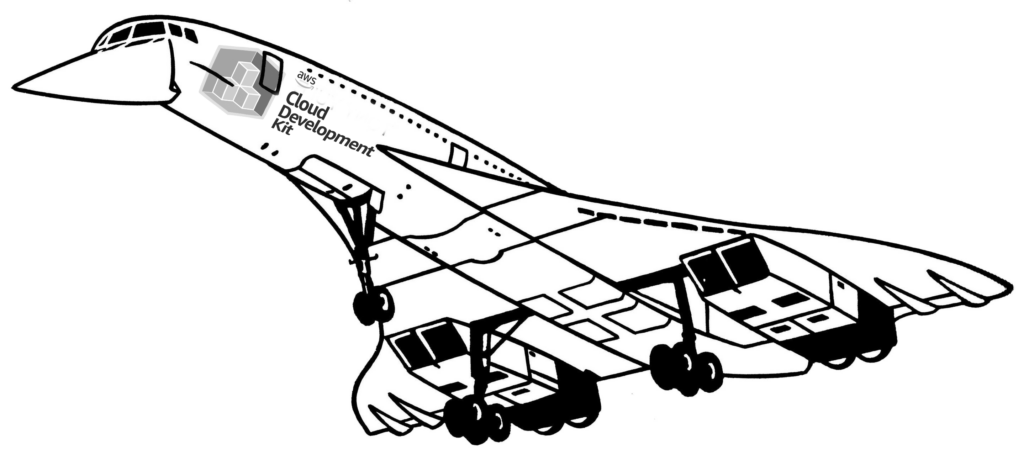
In CDK, Stacks are declared as Class-level variables. Stacks can be nested or deployed side-by-side. Stacks can share resources using the native object-oriented encapsulation quality of class-based Typescript. Stacks can be encapsulated in Typescript classes, and provide access to other Stack classes through public variables. For example and S3 bucket declared in stack A can be exposed as a public class variable which can be passed into the constructor of a different class, which may declare stack B. Resources can be defined abstractly; only the mandatory attributes need be declared, although all parameters configurable through the console are also available to be set through CDK Constructs.
Resources are defined as “constructs” in CDK which are well-documented and provide the same programmatic access to properties as the same resources provisioned with the AWS console. Additionally, CDK provides higher-level construct which allows multiple cloud resources to be specified by a single CDK construct. For example Level 2 VPC constructs can deploy a complete VPC with resource sets which include route tables, subnets with ip ranges along with options for public, private or egress-only routing. Level 3 Constructs contain complete applications are available in the AWS Construct Library.
CDK Pipelines is a handy library which generates self-mutating pipelines for deployment. The pipelines are AWS CodePipeline instances with can source from Github, BitBucket or Gitlab and can be deployed across accounts. A CDK Pipeline can run in a shared services AWS account, and within the same region, deploying into development and production environments hosted in separate accounts. Each deployment is assign a separate stage in the CodePipeline, test suites and manual approval steps can be included.
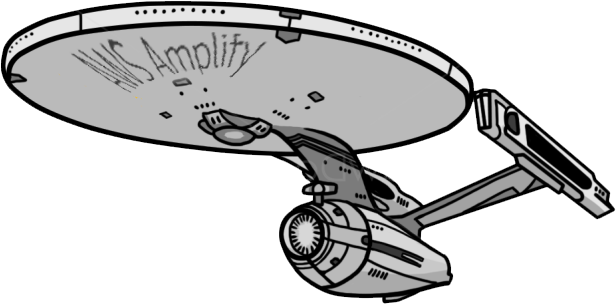
When projects do not require a virtual private cloud or extensive networking configuration or EC2-based resources, there are other viable options. The AWS Serverless Application Model (SAM) allows the user to develop and run AWS Lambda functions and AWS API Gateways locally and deploy them via the command line into the AWS Cloud. The Serverless framework (serverless.com) is a third-party service which facilitates deployments by declaring resources with YAML files. Serverless is often used to deploy AI/ML resources. Both SAM and Serverless generate CloudFormation stacks.
What if your project is a more traditional website? AWS takes building your own to new levels with Amplify Gen 2 – now the recommended method of deploying static sites. Comparable to self-hosting platforms like Squarespace and Wix, Amplify is less prescriptive and allows for advanced options and configurations. Amplify is compatible with React, Next.js, Angular, Vue, Javascript, React Native, Flutter, Android and Swift. Amplify supports DynamoDB as a backend data store with an Object-Relational Mapping (ORM) which links application code to DynamoDB through AppSync. Database Events can be captured within the Amplify code API and propagated to AWS Lambda functions configured through Amplify. Additionally, the new AI Kit for Amplify allows developers to easily integrate Generative AI into their code. Amplify includes it’s own internal CI/CD pipeline and uses CDK constructs to declare resources. Rather than setting up CodePipeline deploying to an S3 bucket for hosting, it is much better to use the Amplify pipeline for deployment; freeing your development time for innovation.
These CI/CD options will continue to evolve, and given a new project, there are so many deployment options, it is hard to choose. Finding the right deployment strategy depends on the resources needed, access patterns, size of day and the code complexity of the project at hand. More and more, deployment is becoming the developer’s responsibility.
0 Comments